Configuring the Module¶
The Gini Vision Library is built with the option to adopt the styling regarding the needs of your CI. Therefore the UI resources used by the Gini Vision Library can be replaced or customized. Furthermore specific options are given to change the behavior of the Gini Vision Library.
Note
All configurations are optional. The Gini Vision Library will work out of the box with no configuration.
Screen Resources¶
You can replace the images used by the library simply by replacing images in the GiniVision.bundle with the exact same resolution and name. Remember to always replace all three variants (@1x, @2x and @3x) of an image. The following screen images will show which image and which text resources are used on which screen. The color scheme is as follows: red- images, blue- texts.
Help Screen #1¶
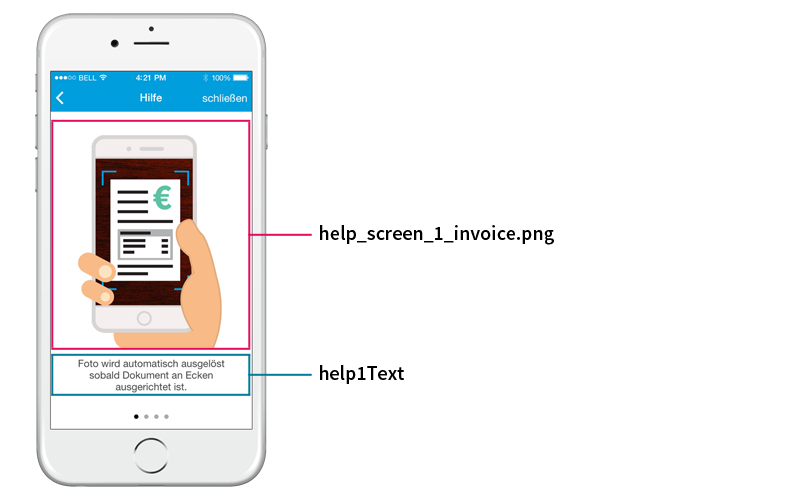
Help Screen #2¶
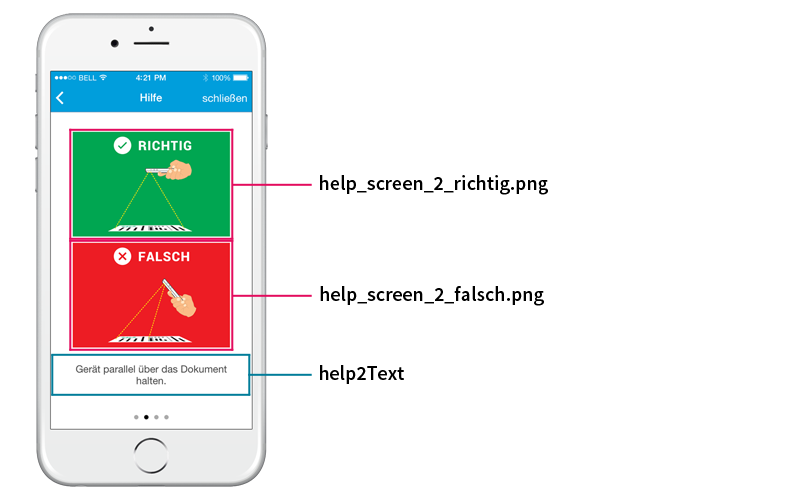
Help Screen #3¶
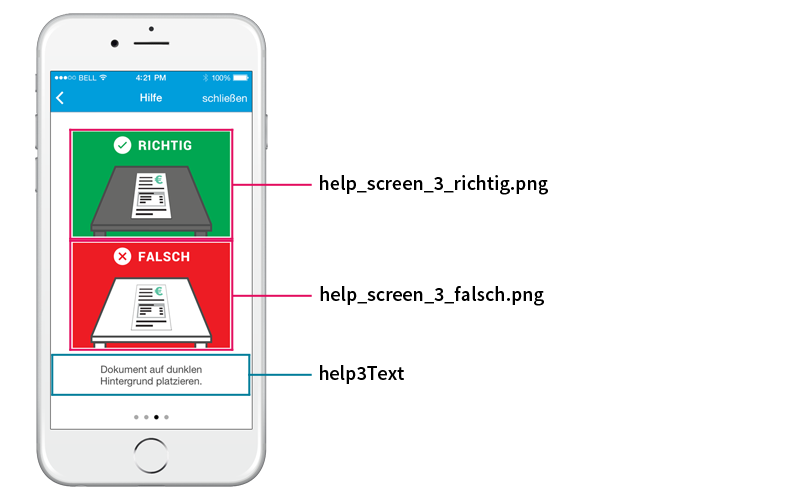
Help Screen #4¶
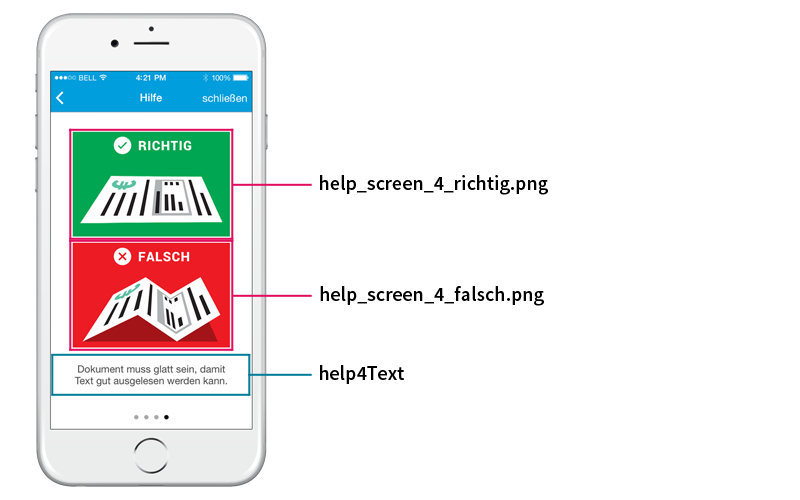
List of all image files¶
- camera_screen_corners_invoice_a4remittance.png corners for invoice or integrated remittance slip.
- camera_screen_corners_remittance.png corners for remittance slip.
- messages_nahe_ran.png move closer message.
- messages_nicht_bewegen.png hold device still message.
- help_screen_1_invoice.png first help image
- help_screen_2_richtig.png second help image (right).
- help_screen_2_falsch.png second help image (wrong).
- help_screen_3_richtig.png third help image (right).
- help_screen_3_falsch.png third help image (wrong).
- help_screen_4_richtig.png fourth help image (right).
- help_screen_4_falsch.png fourth help image (wrong).
Don’t forget to add the respective multiplier at the end of an image file name, e.g. help_screen_1_invoice@2x.png.
List of all text resources¶
Find all configurable text resources in the Texts section.
Use the GiniConfiguration to customize look and behavior of the module¶
To configure colors, fonts and more options the Gini Vision Library provides a GiniConfiguration singleton class. In order to start the scanner with your own configuration call captureImageWithViewController:delegate:configuration: on the GiniVision class. The following properties can be configured.
Options¶
- launchDocumentType of type GINIDocumentType skips the choice screen if it is different from GINIDocumentTypeDefault and starts the scanner with the provided document type.
- showHelp of type BOOL shows the help screen directly after starting the Gini Vision Library.
- disableInteractiveSwipeGesture of type BOOL disables swipe back gesture on navigation controller.
- createJPEGDocumentWithMetaData of type BOOL additionally returns a data object containing the image and meta information about the image.
- navigationShadowImage of type UIImage sets an image for the navigation bar’s shadow. You can use the [GiniConfiguration imageWithColor:andSize:] helper method to create a monochrome shadow image. Can be used for example to create a one pixel thick line below the navigation bar.
- navigationBackButtonImage of type UIImage sets the background image for the navigation bar’s back button.
- choiceHeadingTextSize of type CGFloat sets the text size of the heading text in the choice screen.
- outlineWidth of type CGFloat sets the width of the document outline shown in the camera viewfinder.
- helpButtonImage of type UIImage sets an image for the help button in the scanner’s UINavigationBar.
- helpCloseButtonPosition of type GINIHelpCloseButtonPosition sets the position of the close button in the help screen to the right or the left side.
- helpCloseButtonRemoveSpacing of type BOOL removes the spacing around the close button. Helpful when the button is on the left side to align it correctly with the other close buttons.
- closeButtonImage of type UIImage sets an image for the close button. If image is not set text “Schließen” will be used instead.
- uploadActivityIndicatorPosition of type GINIUploadActivityIndicatorPosition sets the position of the activity indicator in the upload screen.
- customActivityIndicatorImage of type UIImage sets the image which will be used for the custom activity indicator. This image will be shown rotating centered on the preview image in the upload screen. uploadActivityIndicatorPosition must be set to GINIUploadActivityIndicatorPositionImageCenterCustom for the image to be used.
Colors¶
All colors are of type UIColor.
- navigationTintColor sets tint color of the navigation bar and the background of all regular buttons.
- navigationButtonsTintColor sets the tint color of the navigation bar default buttons.
- lightFontColor sets light font color.
- darkFontColor sets dark font color, it is used for regular text.
- actionFontColor will be used in the future to highlight specific text.
- backgroundColor sets the background color for all screens except the help screens.
- outlineColor sets the color of the rectangular overlay of the camera view.
- helpViewBackgroundColor sets the background color in the help screens.
- uploadCancelButtonBackgroundColor sets the background color for the cancel button in the upload screen. If not set navigationTintColor is used.
Fonts¶
All fonts are of type UIFont. Therefore size and font family can be set.
- navigationFont sets font for all elements in the navigation bar.
- textFont sets font for regular text e.g. text below help images.
- buttonFont sets font for regular button titles.
Note
To use the default iOS system font set the font attribute to nil.
Texts¶
All texts are of type NSString.
- choiceTitle sets title text for the choice view controller.
- scannerTitle sets the default title text for the scanner view controller.
- scannerTitleInvoice sets the title text for the scanner view controller, when the document type is invoice. If not set scannerTitle is used.
- scannerTitleRemittance sets the title text for the scanner view controller, when the document type is remittance. If not set scannerTitle is used.
- scannerTitleIntegratedRemittance sets the title text for the scanner view controller, when the document type is integrated remittance. If not set scannerTitle is used.
- helpTitle sets title text for help view controller.
- uploadTitle sets title text for upload view controller.
- choiceHeadingText sets text for choice view controller heading.
- help1Text sets text for help view controller text 1.
- help2Text sets text for help view controller text 2.
- help3Text sets text for help view controller text 3.
- help4Text sets text for help view controller text 4.
- uploadCancelButtonTitle sets text for upload view controller cancel button title.
- cameraAuthErrorTitle sets error title for failed camera authorization.
- cameraAuthErrorText sets error text for failed camera authorization.
Example¶
The example considers the same structure shown previously. It shows all possible configurations and their default values.
//
// MyViewController.m
//
- (IBAction)scanDocument:(UIButton *)sender {
GiniConfiguration *configuration = [GiniConfiguration new];
// Options
configuration.launchDocumentType = GINIDocumentTypeDefault; // Remember 'GINIDocumentTypeDefault' does NOT skip the choice screen.
configuration.showHelp = NO;
configuration.disableInteractiveSwipeGesture = NO;
configuration.createJPEGDocumentWithMetaData = NO;
configuration.navigationShadowImage = nil;
configuration.navigationBackButtonImage = nil;
configuration.choiceHeadingTextSize = 34.0;
configuration.outlineWidth = 5.0;
configuration.helpButtonImage = nil;
configuration.helpCloseButtonPosition = GINIHelpCloseButtonPositionNavBarRight;
configuration.helpCloseButtonRemoveSpacing = NO;
configuration.closeButtonImage = nil;
configuration.uploadActivityIndicatorPosition = GINIUploadActivityIndicatorPositionNavBarLeft;
configuration.customActivityIndicatorImage = nil;
// Colors
configuration.navigationTintColor = [UIColor colorWithRed:(28/255.0) green:(141/255.0) blue:(214/255.0) alpha:1.0];
configuration.navigationButtonsTintColor = nil;
configuration.lightFontColor = [UIColor colorWithRed:(255/255.0) green:(255/255.0) blue:(255/255.0) alpha:1.0];
configuration.darkFontColor = [UIColor colorWithRed:(76/255.0) green:(76/255.0) blue:(76/255.0) alpha:1.0];
configuration.actionFontColor = [UIColor colorWithRed:(28/255.0) green:(141/255.0) blue:(214/255.0) alpha:1.0];
configuration.backgroundColor = [UIColor colorWithRed:(255/255.0) green:(255/255.0) blue:(255/255.0) alpha:1.0];
configuration.outlineColor = [UIColor colorWithRed:(0/255.0) green:(255/255.0) blue:(0/255.0) alpha:1.0];
configuration.helpViewBackgroundColor = [UIColor colorWithRed:(255/255.0) green:(255/255.0) blue:(255/255.0) alpha:1.0];
configuration.uploadCancelButtonBackgroundColor = [UIColor colorWithRed:(28/255.0) green:(141/255.0) blue:(214/255.0) alpha:1.0];
// Fonts
configuration.navigationFont = [UIFont fontWithName:@"HelveticaNeue-Light" size:16.0];
configuration.textFont = [UIFont fontWithName:@"HelveticaNeue" size:16.0];
configuration.buttonFont = [UIFont fontWithName:@"HelveticaNeue-Bold" size:16.0];
// Texts
configuration.choiceTitle = @"Foto-Überweisung";
configuration.scannerTitle = @"Foto-Überweisung";
configuration.scannerTitleInvoice = @"Foto-Überweisung";
configuration.scannerTitleRemittance = @"Foto-Überweisung";
configuration.scannerTitleIntegratedRemittance = @"Foto-Überweisung";
configuration.helpTitle = @"Hilfe";
configuration.uploadTitle = @"Foto-Überweisung";
configuration.choiceHeadingText = @"Welcher Dokument-Typ?";
configuration.help1Text = @"Foto wird automatisch ausgelöst sobald Dokument an Ecken ausgerichtet ist.";
configuration.help2Text = @"Gerät parallel über das Dokument halten.";
configuration.help3Text = @"Dokument auf dunklen Hintergrund platzieren.";
configuration.help4Text = @"Dokument muss glatt sein, damit Text gut ausgelesen werden kann.";
configuration.uploadCancelButtonTitle = @"Abbrechen";
configuration.cameraAuthErrorTitle = @"Kein Kamerazugriff erlaubt";
configuration.cameraAuthErrorText = @"Bitte aktivieren Sie unter Einstellungen > Datenschutz > Kamera die Zugriffsrechte für diese Anwendung.";
// Call Gini Vision Library with configuration
[GiniVision captureImageWithViewController:self delegate:self configuration:configuration];
}
//
// MyViewController.swift
//
@IBAction
func scanDocument(sender: AnyObject) {
let configuration = GiniConfiguration()
// Options
configuration.launchDocumentType = .Default // Remember 'GINIDocumentTypeDefault' does NOT skip the choice screen.
configuration.showHelp = false
configuration.disableInteractiveSwipeGesture = false
configuration.createJPEGDocumentWithMetaData = false
configuration.navigationShadowImage = nil
configuration.navigationBackButtonImage = nil
configuration.choiceHeadingTextSize = 34.0
configuration.outlineWidth = 5.0
configuration.helpButtonImage = nil
configuration.helpCloseButtonPosition = .NavBarRight
configuration.helpCloseButtonRemoveSpacing = false
configuration.closeButtonImage = nil
configuration.uploadActivityIndicatorPosition = .NavBarLeft
configuration.customActivityIndicatorImage = nil
// Colors
configuration.navigationTintColor = UIColor(red: (28/255.0), green: (141/255.0), blue: (214/255.0), alpha: 1.0)
configuration.navigationButtonsTintColor = nil
configuration.lightFontColor = UIColor(red: (255/255.0), green: (255/255.0), blue: (255/255.0), alpha: 1.0)
configuration.darkFontColor = UIColor(red: (76/255.0), green: (76/255.0), blue: (76/255.0), alpha: 1.0)
configuration.actionFontColor = UIColor(red: (28/255.0), green: (141/255.0), blue: (214/255.0), alpha: 1.0)
configuration.backgroundColor = UIColor(red: (255/255.0), green: (255/255.0), blue: (255/255.0), alpha: 1.0)
configuration.outlineColor = UIColor(red: (0/255.0), green: (255/255.0), blue: (0/255.0), alpha: 1.0)
configuration.helpViewBackgroundColor = UIColor(red: (255/255.0), green: (255/255.0), blue: (255/255.0), alpha: 1.0)
configuration.uploadCancelButtonBackgroundColor = UIColor(red: (28/255.0), green: (141/255.0), blue: (214/255.0), alpha: 1.0)
// Fonts
configuration.navigationFont = UIFont(name: "HelveticaNeue-Light", size: 16.0)
configuration.textFont = UIFont(name: "HelveticaNeue", size: 16.0)
configuration.buttonFont = UIFont(name: "HelveticaNeue-Bold", size: 16.0)
// Texts
configuration.choiceTitle = "Foto-Überweisung"
configuration.scannerTitle = "Foto-Überweisung"
configuration.scannerTitleInvoice = "Foto-Überweisung"
configuration.scannerTitleRemittance = "Foto-Überweisung"
configuration.scannerTitleIntegratedRemittance = "Foto-Überweisung"
configuration.helpTitle = "Hilfe"
configuration.uploadTitle = "Foto-Überweisung"
configuration.choiceHeadingText = "Welcher Dokument-Typ?"
configuration.help1Text = "Foto wird automatisch ausgelöst sobald Dokument an Ecken ausgerichtet ist."
configuration.help2Text = "Gerät parallel über das Dokument halten."
configuration.help3Text = "Dokument auf dunklen Hintergrund platzieren."
configuration.help4Text = "Dokument muss glatt sein, damit Text gut ausgelesen werden kann."
configuration.uploadCancelButtonTitle = "Abbrechen"
configuration.cameraAuthErrorTitle = "Kein Kamerazugriff erlaubt"
configuration.cameraAuthErrorText = "Bitte aktivieren Sie unter Einstellungen > Datenschutz > Kamera die Zugriffsrechte für diese Anwendung."
GiniVision.captureImageWithViewController(self, delegate: self, configuration: configuration)
}